Master Python Regex Testing
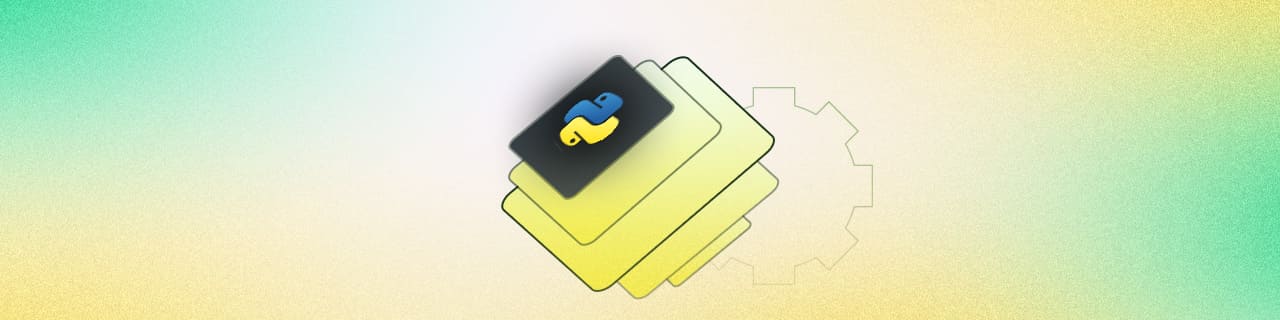
Accuracy and efficacy are critical pillars in the field of automation testing. Regular Expressions, sometimes known as regex, emerges as a strong tool capable of taking your testing toolset to new heights. Python is a versatile and strong programming language, and one of its primary strengths is its support for regular expressions, sometimes known as regex.
This blog explains Python regex testing, highlighting its importance in many automation applications. We will demonstrate its practical use through hands-on examples, supplemented by crucial libraries that ease and empower the process.
What is Python Regex?
Regular expressions, or regex, are character sequences that define a search pattern. This pattern can be used to match, locate, and manipulate text, providing a powerful tool for string manipulation. Whether you need to validate email addresses, extract specific information from a text, or replace substrings, regex is your ally.
Key Points:
- Flexible Pattern Matching: Regex can identify and match specific patterns within text, enabling tasks like finding email addresses or phone numbers.
- Efficient String Validation: Validate formats such as email addresses, phone numbers, and zip codes with high precision.
- Effective Text Extraction: Extract pertinent data from strings, such as webpage URLs or text dates.
- Powerful Text Replacement: Find and replace text efficiently, useful in data sanitization and reformatting tasks.
- Advanced Search Patterns: Supports intricate search patterns, simplifying complex string manipulations.
- Seamless Python Integration: Python’s built-in re module offers comprehensive functions for regex, making it an integral part of both simple scripts and complex automation projects.
How to Use Regex in Python?
Leveraging Python's built-in re-module is essential for unleashing the complete power of regex in the language. This module equips developers with a suite of functions, seamlessly facilitating the integration of regular expressions into various aspects of test automation.
Addressing tasks ranging from straightforward pattern matching to intricate string manipulations, Python's regex capabilities provide developers with the tools needed to handle diverse challenges in the realm of test automation.
How to Use Regular Expression in Python with Examples?
Mastering regex components in the vast landscape of Python programming provides a powerful arsenal for automation testers. Let's delve into these components with practical examples, showcasing how they can be combined to perform versatile string manipulations and pattern matching.
Anchors (^ and $):
Anchors define the start and end positions of a match within a string. For instance:
import re pattern = r'^Hello' text = 'Hello, Tester!' match = re.search(pattern, text)
Here, the pattern '^Hello' ensures that the match occurs only at the beginning of the string.
Character Classes ([ ]):
Character classes specify sets of characters that can match at a certain position. Example:
pattern = r'[aeiou]' text = 'Hello' match = re.search(pattern, text)
This pattern matches any vowel in the string 'Hello'.
Quantifiers (*, +, ?, { }):
Quantifiers determine the number of occurrences of a character or group. Example:
pattern = r'\d{3}-\d{2}-\d{4}' text = '123-45-6789' match = re.search(pattern, text)
This pattern matches a social security number in the format '123-45-6789'.
Escape Sequences ():
Escape sequences match special characters literally. Example:
pattern = r'\$50' text = 'The price is $50' match = re.search(pattern, text)
This pattern matches the exact occurrence of '$50' in the text.
Alternation (|):
Alternation allows selecting from multiple alternatives. Example:
pattern = r'cat|dog' text = 'I have a cat' match = re.search(pattern, text)
This pattern matches either 'cat' or 'dog' in the string.
Groups and Capturing (( )):
Groups create subexpressions for complex patterns. Example:
pattern = r'(\d{2})-(\d{2})-(\d{4})' text = 'Date: 01-01-2023' match = re.search(pattern, text) day, month, year = match.groups()
This pattern captures and extracts the day, month, and year from a date string.
Character Escapes (\d, \w, \s, etc.):
Character escapes provide shortcuts for character classes. Example:
pattern = r'\d{3}\s\w+' text = '123 John' match = re.search(pattern, text)
This pattern matches a three-digit number followed by a space and a word.
Lazy Matching (*?, +?, ??, { }?):
Lazy matching performs minimal matches. Example:
pattern = r'<.*?>' text = 'Paragraph 1
Paragraph 2
' match = re.search(pattern, text)
This pattern performs a non-greedy match, capturing each individual paragraph.
Assertions ((?= ) and (?! )):
Assertions enforce conditions on patterns. Example:
pattern = r'\bword\b(?= is)' text = 'This word is valuable.' match = re.search(pattern, text)
This pattern matches the word 'word' only if it is followed by ' is'.
Backreferences (\1, \2, etc.):
Backreferences reference and match previously captured groups. Example:
pattern = r'(\d{2})--\d{4}' text = '22-22-2022' match = re.search(pattern, text)
This pattern matches a date with repeated day and month values.
Flags (re.IGNORECASE, re.DOTALL, etc.):
Flags modify regex behavior. Example:
pattern = r'case-insensitive' text = 'Case-Insensitive' match = re.search(pattern, text, re.IGNORECASE)
This pattern matches 'case-insensitive' regardless of case.
Guide to Python Regex Testing: A Precise Approach
Python regex testing is a methodical process for validating and manipulating strings based on specified patterns. Follow these precise steps to conduct effective regex testing:
Step 1: Import the re Module
To utilize regex in Python, initiate the process by importing the built-in re module:
import re
Step 2: Define the Regex Pattern
Clearly articulate the pattern you aim to match or search for within a string. Regular expressions are expressed as strings; thus, define your regex pattern accordingly.
Step 3: Choose the Appropriate Function
Select the suitable re function based on your objective:
- re.match(): Verifies if the pattern matches at the beginning of the string.
- re.search(): Scans the entire string for a match.
- re.findall(): Retrieves all occurrences of the pattern in the string.
- re.finditer(): Yields an iterator of match objects for all occurrences.
- re.sub(): Substitutes the pattern with a replacement string.
Step 4: Apply the Regex Function
Execute the chosen re function alongside the regex pattern and the target string to perform the desired operation. Capture the result in a variable if necessary.
Step 5: Process the Results
Tailor your approach based on the function used:
- For re.match(), re.search(), and re.finditer(), interact with the returned match object to access matched text and groups.
- For re.findall(), obtain a list of matched substrings.
- For re.sub(), the function replaces matches with the specified replacement.
Real time Examples for Regex with Python
These examples showcase the application of Python regex in test automation, from validating email addresses to extracting information like URLs and Social Security Numbers. Utilizing regex in these contexts enhances the precision and efficiency of test automation processes.
Example: Email Address Validation
Ensure an email address follows a standard format by employing Python regex for validation:
import re email_pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$' email = "sidharthsdet@accelq.com" if re.match(email_pattern, email): print("Valid email address") else: print("Invalid email address")
Example: Extracting Hyperlinks
If your objective is to extract URLs from text, utilize re.findall() as illustrated below:
import re text = "Explore our website at https://www.accelq.com for more information." url_pattern = r'https?://\S+' urls = re.findall(url_pattern, text) print(urls)
Example: Validating and Extracting Social Security Numbers
Consider a scenario where users input their Social Security Numbers on a form. Validate the format and extract them for further processing using Python regex:
import re # Sample text with Social Security Numbers text = "Submit your details: 321-95-7657 and 837462543" # Define the regex pattern for Social Security Numbers ssn_pattern = r'\b\d{3}-\d{2}-\d{4}\b|\b\d{9}\b' # Find all matches using `re.findall()` ssn_list = re.findall(ssn_pattern, text) # Validate and print the extracted Social Security Numbers for ssn in ssn_list: print(f"Valid Social Security Number: {ssn}")
In this scenario, the ssn_pattern regex accommodates two formats: ###-##-#### and #########, where # represents a digit. The \b word boundary ensures complete matching of Social Security Numbers. The re.findall() function extracts all matched numbers, and the subsequent loop validates and prints the results.
Python Third-Party Libraries for Regex Testing:
1. regex Library:
Description: The regex library is an advanced regex implementation with additional features compared to the built-in re module. It supports Unicode properties and provides powerful functionalities.
2. re2 Library:
Description: The re2 library is a Python binding for Google's RE2 test a regular expression in python library. It is designed for efficiency and provides linear-time matching on all inputs.
3. regex-dfa Library:
Description: This library implements regular expression matching using deterministic finite automata (DFA). It can be more efficient than backtracking-based approaches for certain patterns.
4. pyre-check Library:
Description: Pyre-check is a static type checker for Python, helping catch type-related issues in your code. While not specifically a regex library, it can assist in identifying type-related issues in regex patterns.
Conclusion
Mastering Python regex testing is a valuable skill that can significantly enhance your endeavors in automation testing. Regex provides a versatile and impactful solution for tasks ranging from data validation to log parsing. By becoming proficient in Python regex and leveraging tools like re and regex, you can create automation tests that are not only more reliable but also more efficient. Step into the realm of regex to elevate the precision of your automation testing to new heights.
Balbodh Jha
Associate Director Product Engineering
Balbodh is a passionate enthusiast of Test Automation, constantly seeking opportunities to tackle real-world challenges in this field. He possesses an insatiable curiosity for engaging in discussions on testing-related topics and crafting solutions to address them. He has a wealth of experience in establishing Test Centers of Excellence (TCoE) for a diverse range of clients he has collaborated with.
Discover More
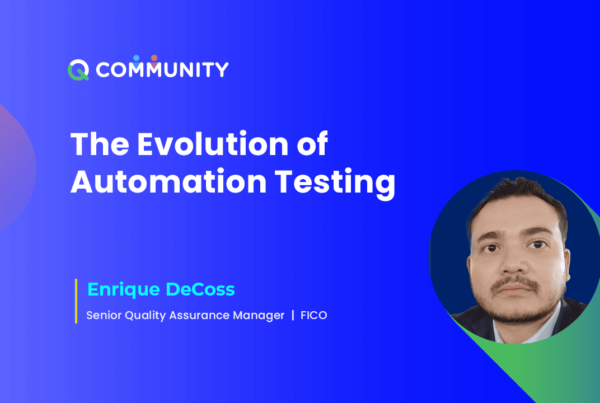
The Evolution of Automation Testing
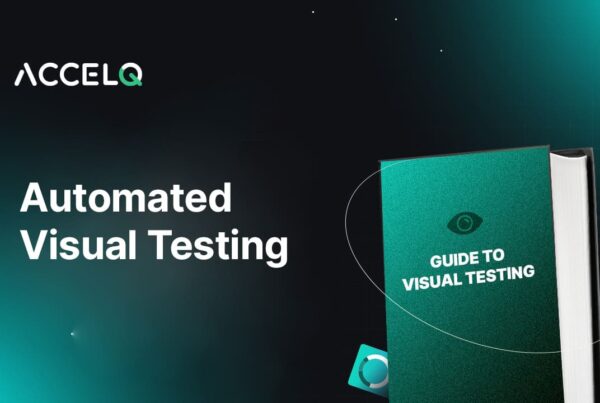